feign是一个声明式WebService客户端。使用Feign能让编写Web Service客户端更加简单。它的使用方法是定义一个服务接口然后在上面添加注解。Feign也支持可拔插式的编码器和解码器。Spring Cloud对Feign进行封装,使其支持了Spring MVC标准注解和HttpMessageConverters。Feign可以与Eureka和Ribbon组合使用支持负载均衡。
Feign集成了Ribbon,利用Ribbon维护了Payment的服务列表信息,并且通过轮询实现了客户端的负载均衡,而与Ribbon不同的是,通过Feign值需要定义服务绑定接口且一声明式的方法,优雅而简单的实现了服务调用。
feign和openFeign有什么区别?
Feign | OpenFeign |
Feign是Spring Cloud组件中一个轻量级RESTful的HTTP服务客户端。Feign内置了Ribbon,用来做客户端负载均衡,去调用服务注册中心的服务。 | OpenFeign是Spring Cloud在Feign的基础上支持了Spring MVC,如@RequestMapping等。 |
<!--feign-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
</dependency>
<!--openfeign-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
本文使用Feign进行创建。
我们前面的程序架构是这样:
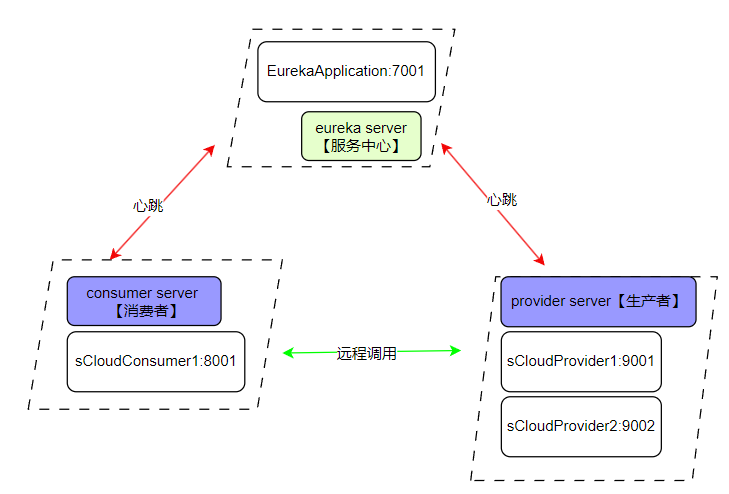
这篇文章增加一个消费者,用feigin进行创建,创建后的框架参考如下:
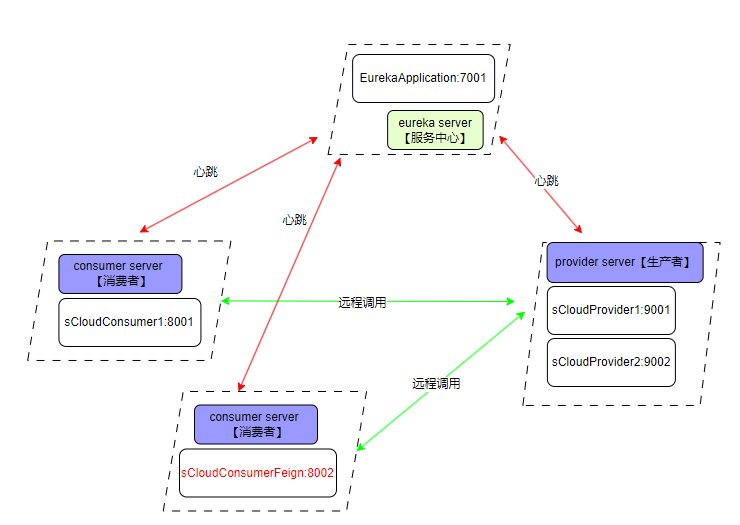
1.创建feign的module
右击父工程,创建一个module,
POM:
代码版本可以去:https://mvnrepository.com/
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>s-cloud</artifactId>
<groupId>site.longkui</groupId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>s-cloud-comsumer-feign</artifactId>
<dependencies>
<!-- boot-web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- eureka 客户端 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
<version>3.1.8-SNAPSHOT</version>
</dependency>
<!-- openfegin -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<version>3.1.8</version>
</dependency>
</dependencies>
</project>
然后在这个module下面创建controller和service文件夹
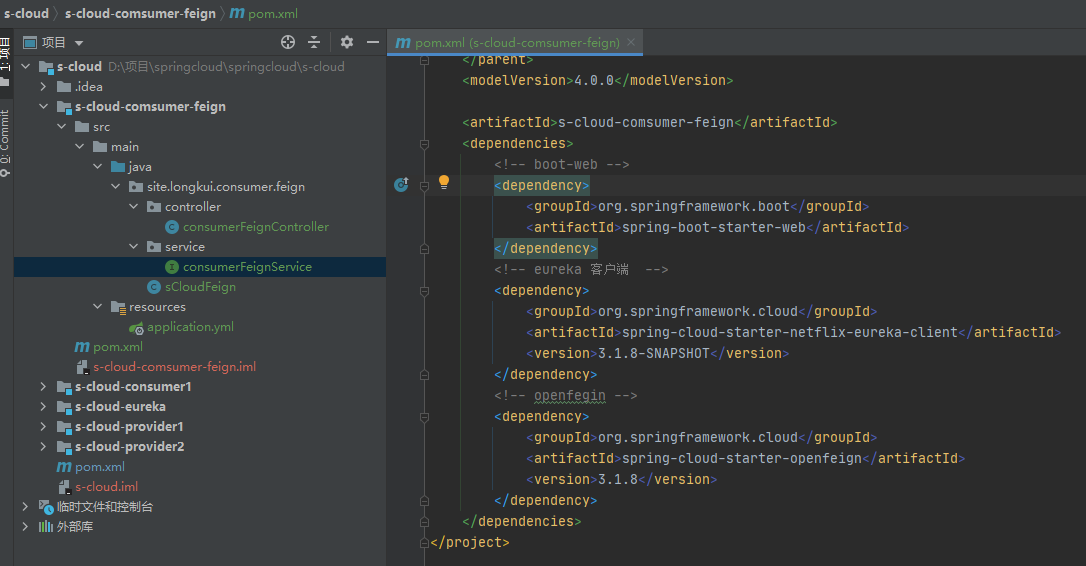
编写配置文件,application.yml
server:
port: 8002 #服务的端口
#spring相关配置
spring:
application:
name: service-consumer-feign #服务的名称
#eureka配置
eureka:
client:
service-url:
# 填写注册中心服务器地址
defaultZone: http://localhost:7001/eureka
# 是否需要将自己注册到注册中心
register-with-eureka: true
# 是否需要搜索服务信息
fetch-registry: true
instance:
# 使用ip地址注册到注册中心
prefer-ip-address: true
# 注册中心列表中显示的状态参数
instance-id: ${spring.cloud.client.ip-address}:${server.port}
修改主启动类:
package site.longkui.consumer.feign;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
import site.longkui.consumer.feign.service.consumerFeignService;
@SpringBootApplication
@EnableEurekaClient //Eureka客户端
@EnableFeignClients//开启feign
public class sCloudFeign {
public static void main(String[] args) {
SpringApplication.run(sCloudFeign.class, args);
}
}
创建service: consumerFeignService,这里要和生产者(服务提供者)的路由参数都一样,@FeignClient(“service-provider1”)表示需要访问哪个服务
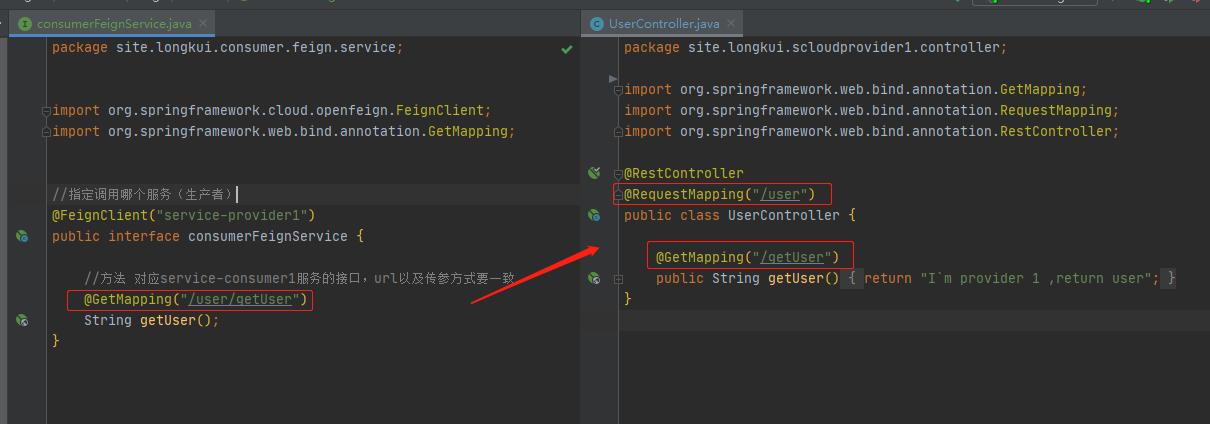
创建controller,来访问刚才创建的service
package site.longkui.consumer.feign.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import site.longkui.consumer.feign.service.consumerFeignService;
@RestController
@RequestMapping("/consumerFeign")
public class consumerFeignController {
@Autowired
private consumerFeignService feignService;
@GetMapping("/getUser")
public String getUser(){
return feignService.getUser();
}
}
然后启动项目,首先看看服务有没有上线:
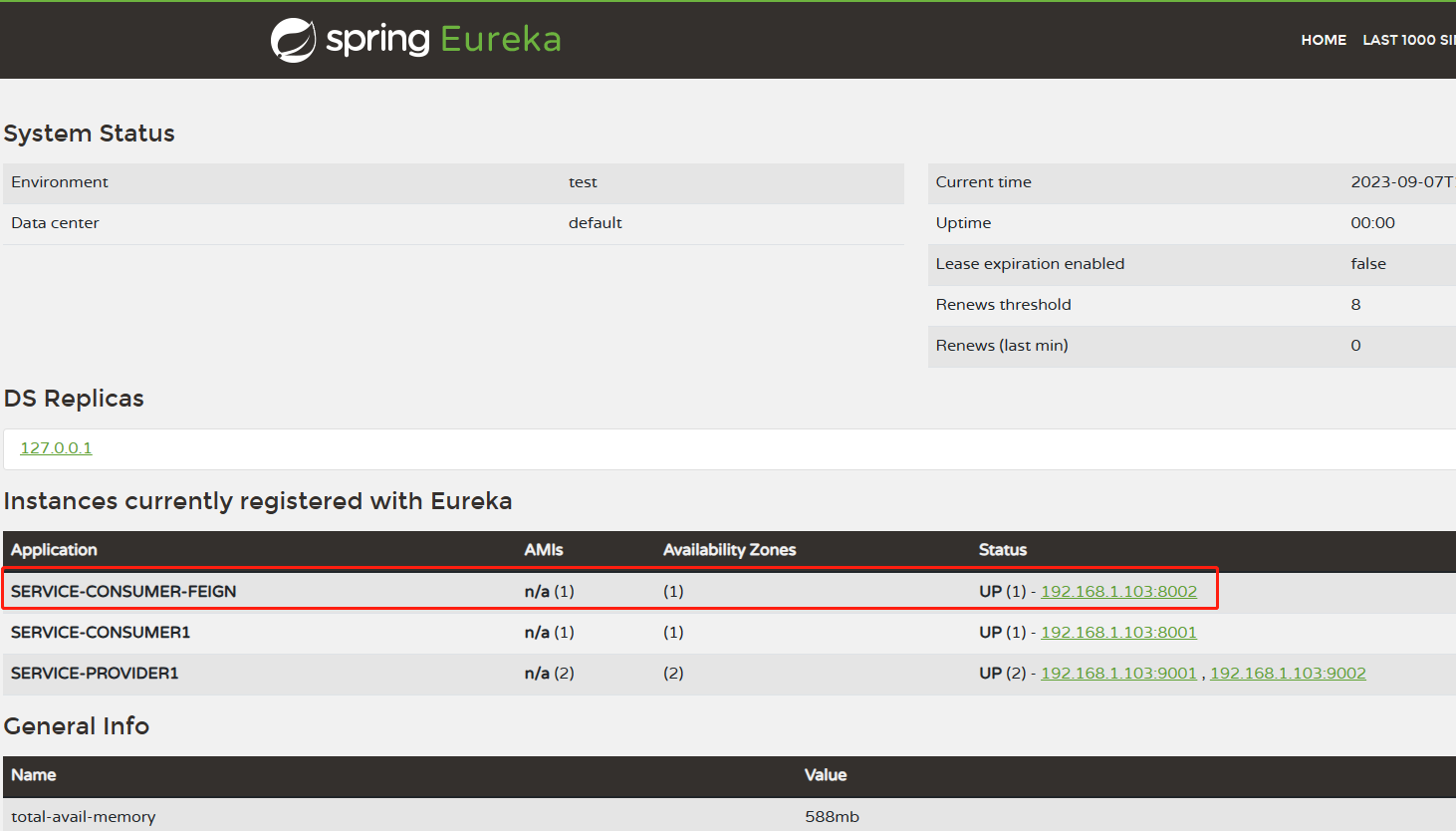
然后访问支持feign的消费者

可以看到,正常访问,而且实现了负载均衡。